1. org.apache.commons.lang3.tuple 包
1.1 抽象类 Pair (二元组)
- Pair 是抽象类,不可直接实例化,通过 Pair.of(L,R) 实例化
- Pair 只放一组 key/value,在接口需返回两个值时比较适用
public abstract class Pair implements Map.Entry, Comparable>, Serializable {
public static Pair of(final L left, final R right) {
return ImmutablePair.of(left, right);
}
public final L getKey() {
return getLeft();
}
public R getValue() {
return getRight();
}
}
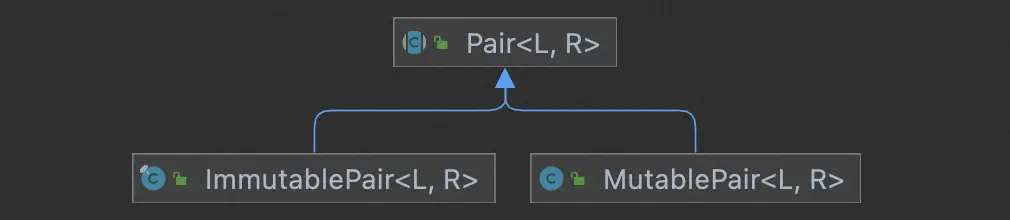
public final class ImmutablePair extends Pair {
@Override
public R setValue(final R value) {
throw new UnsupportedOperationException();
}
}
public class MutablePair extends Pair {
}
1.2 抽象类 Triple (三元组)
public abstract class Triple implements Comparable>, Serializable {
public static Triple of(final L left, final M middle, final R right) {
return new ImmutableTriple(left, middle, right);
}
}
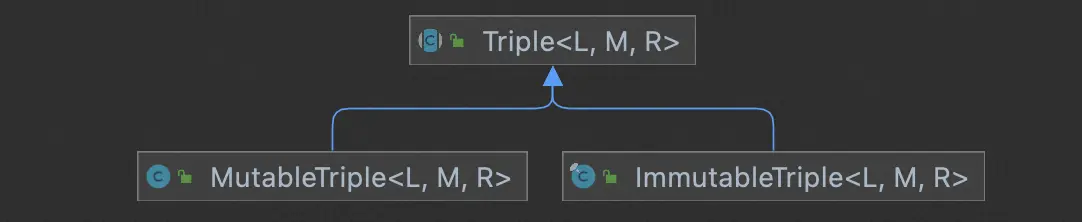
2. Pair 示例
2.1 示例
@Test
public void isMutable() {
// 不可变配对
Pair pair = new ImmutablePair("left", "right");
// 可变配对
Pair mutablePair = new MutablePair("left", "right");
// pair.setValue("333"); // java.lang.UnsupportedOperationException
mutablePair.setValue("111");
// (left,right); (left,111)
System.out.println(pair + "; " + mutablePair);
}
@Test
public void demo() {
Pair pair = Pair.of("left", "right");
System.out.println(pair.getKey() + "; " + pair.getLeft());
System.out.println(pair.getValue() + "; " + pair.getRight());
// setNull
Pair pair2 = Pair.of(null, null);
Pair pair3 = Pair.of(null, "right");
Pair pair4 = Pair.of("left", null);
System.out.println(pair2 + "; " + pair3 + "; " + pair4);
}
2.2 结合Map
@Test
public void combineMap() {
Map> map = new HashMap();
map.put(1001L, Pair.of("left", "right"));
Map, Boolean> booleanMap = new HashMap();
booleanMap.put(Pair.of("left", "right"), true);
}
3. Triple 示例
@Test
public void triple() {
Triple triple = Triple.of("left", "middle", "right");
System.out.println(triple.getLeft() + "; " + triple.getMiddle() + "; " + triple.getRight());
Triple, String, List> multiTriple =
Triple.of(Pair.of("userId", "orderId"), "middle", Lists.newArrayList("right1", "right1"));
System.out.println(multiTriple);
}
【信息由网络或者个人提供,如有涉及版权请联系COOY资源网邮箱处理】
© 版权声明
部分内容为互联网分享,若有侵权请联系站长删除。
THE END
暂无评论内容