1. Spring Boot 集成 Redis
1.1 引入依赖
org.springframework.boot spring-boot-starter-data-redisorg.apache.commons commons-pool2
SpringBoot 默认使用 Lettuce,查看 spring-boot-starter-data-redis-2.7.5.pom
org.springframework.boot spring-boot-starter
...
io.lettuce lettuce-core
...
1.2 配置 application.yml
spring:
redis:
database: 0
host: 127.0.0.1
port: 6379
password: admin
lettuce:
pool:
max-idle: 16
max-active: 32
min-idle: 8
1.3 启动 Redis
# redis 位置
cd /Users/.../redis-7.0.5
# 启动
redis-server
# 客户端
redis-cli
# 关闭客户端
redis-cli shutdown
1.4 其他
若要使用 jedis,需要排除lettuce
redis.clients jedis4.2.3
spring:
redis:
...
jedis:
pool:
max-active: 8
max-idle: 8
min-idle: 6
2. RedisTemplate 工具类
2.1 序列化问题
查看key值,出现无意义的乱码前缀
127.0.0.1:6379> keys *
1) "xacxedx00x05tx00x04name"
原因是 RedisTemplate 默认使用 JdkSerializationRedisSerializer 序列化器
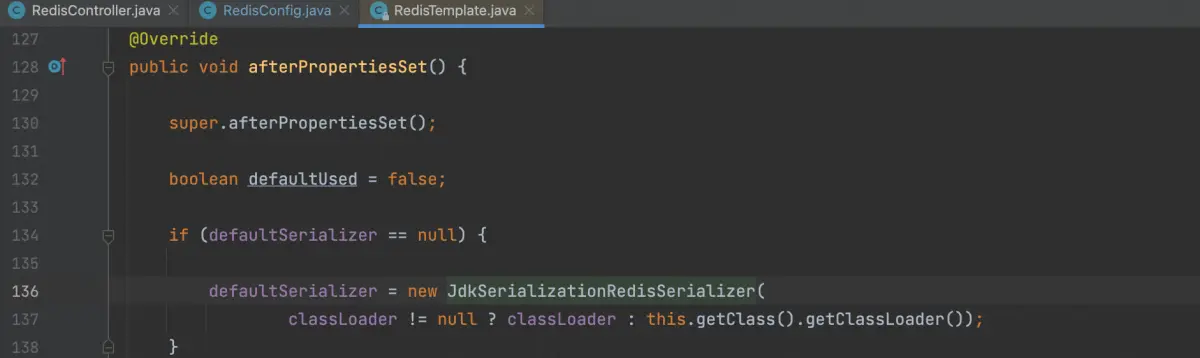
方式一:使用 StringRedisTemplate
@Resource
private StringRedisTemplate stringRedisTemplate;
方式二:更改 RedisTemplate 序列化方式
@RestController
@RequestMapping("/redis")
public class RedisController {
@Resource
private RedisTemplate redisTemplate;
}
@Configuration
public class RedisConfig {
@Bean(name = "redisTemplate")
public RedisTemplate getRedisTemplate(RedisConnectionFactory factory) {
RedisTemplate redisTemplate = new RedisTemplate();
redisTemplate.setConnectionFactory(factory);
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
redisTemplate.setKeySerializer(stringRedisSerializer);
redisTemplate.setValueSerializer(stringRedisSerializer);
redisTemplate.afterPropertiesSet();
return redisTemplate;
}
}
2.2 设置 RedisTemplate 序列化方式升级
@Bean(name = "redisTemplate")
public RedisTemplate getRedisTemplate(RedisConnectionFactory factory) {
RedisTemplate redisTemplate = new RedisTemplate();
redisTemplate.setConnectionFactory(factory);
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
redisTemplate.setKeySerializer(stringRedisSerializer);
redisTemplate.setValueSerializer(jacksonSerializer());
redisTemplate.setHashKeySerializer(stringRedisSerializer);
redisTemplate.setHashValueSerializer(jacksonSerializer());
redisTemplate.afterPropertiesSet();
return redisTemplate;
}
private Jackson2JsonRedisSerializer jacksonSerializer() {
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
objectMapper.activateDefaultTyping(LaissezFaireSubTypeValidator.instance,
ObjectMapper.DefaultTyping.NON_FINAL, JsonTypeInfo.As.PROPERTY);
jackson2JsonRedisSerializer.setObjectMapper(objectMapper);
return jackson2JsonRedisSerializer;
}
3. 实战
3.1 opsForXxx() vs boundXxx()
- opsForXxx()
- 字符串:redisTemplate.opsForValue()
- Hash: redisTemplate.opsForHash()
- list: redisTemplate.opsForList()
- set: redisTemplate.opsForSet()
- 有序set: redisTemplate.opsForZSet()
-
- redisTemplate.boundValueOps()
- redisTemplate.boundHashOps()
- redisTemplate.boundListOps()
- redisTemplate.boundSetOps()
- redisTemplate.boundZSetOps()
@RequestMapping("/opsAndBound")
public String opsAndBound(String key) {
// 获取一个operator (未指定操作对象),在一个连接内可以操作多个 key 以及对应的value
ValueOperations valueOperations = redisTemplate.opsForValue();
Object obj = valueOperations.get(key);
// 获取指定操作对象(key)的 operator, 在一个连接内只能操作这个 key 对应的value
BoundValueOperations boundValueOps = redisTemplate.boundValueOps(key);
Object obj1 = boundValueOps.get();
return obj.toString();
}
3.2 获取 key 相关信息
/**
* http://localhost:8080/redis/all
* http://localhost:8080/redis/delete/all
*
* @return
*/
@RequestMapping("/all")
public String getAllKey() {
return JSON.toJSONString(redisTemplate.keys("*"));
}
/**
* http://localhost:8080/redis/all/regex?regex=*bound*
*/
@RequestMapping("/regexKey")
public String getRegexKey(String regex) {
return JSON.toJSONString(redisTemplate.keys(regex));
}
@RequestMapping("/delete")
public Boolean delete(String key) {
return redisTemplate.delete(key);
}
@RequestMapping("/delete/all")
public Long deleteAll(String key) {
Set keys = redisTemplate.keys("*");
return redisTemplate.delete(keys);
}
/**
* 设置过期时间,单位秒
*/
@RequestMapping("/expire")
public Boolean expireSeconds(String key, long timeout) {
return redisTemplate.expire(key, timeout, TimeUnit.SECONDS);
}
/**
* 添加带过期时间的key
*/
@GetMapping("/setExpireValue")
public String setValue(String key, String value, long timeout){
redisTemplate.opsForValue().set(key, value, timeout, TimeUnit.SECONDS);
return "success";
}
/**
* 获取过期时间,单位秒
*/
@RequestMapping("/getExpire")
public Long getExpire(String key, long timeout) {
return redisTemplate.getExpire(key, TimeUnit.SECONDS);
}
/**
* 移除过期时间
*/
@RequestMapping("/persist")
public Boolean persist(String key) {
return redisTemplate.persist(key);
}
3.3 opsForXxx 方式
/**
* http://localhost:8080/redis/setValue?key=owner&value=Tinyspot
* http://localhost:8080/redis/getValue?key=owner
*/
@GetMapping("/setValue")
public String setValue(String key, String value){
redisTemplate.opsForValue().set(key, value);
return "success";
}
@RequestMapping("/getValue")
public String getValue(String key) {
return redisTemplate.opsForValue().get(key).toString();
}
/**
* void put(H key, HK hashKey, HV value);
* hashOperations.putAll(key, Map);
*
* http://localhost:8080/redis/opsForHash?key=books
* Literature
* |- Poetry
* |- Prose
* Art
* |- Music
* |- Photography
*/
@RequestMapping("/opsForHash")
public String opsForHash(String key, String... hashKey) {
HashOperations hashOperations = redisTemplate.opsForHash();
hashOperations.put(key, "Literature", "Poetry");
hashOperations.put(key, "Literature", "Prose");
hashOperations.put(key, "Art", "Music");
hashOperations.put(key, "Art", "Photography");
Set keys = hashOperations.keys(key);
return JSON.toJSONString(keys);
}
@RequestMapping("/opsForHashDelete")
public Long opsForHashDelete(String key, String hashKey) {
// Long delete(H key, Object... hashKeys)
return redisTemplate.opsForHash().delete(key, hashKey);
}
@RequestMapping("/opsForList")
public String opsForList(String key) {
ListOperations listOperations = redisTemplate.opsForList();
listOperations.leftPush(key, "111");
listOperations.leftPush(key, "222");
List range = listOperations.range(key, 0, listOperations.size(key));
return JSON.toJSONString(range);
}
@RequestMapping("/opsForListAll")
public String opsForListAll(String key) {
ListOperations listOperations = redisTemplate.opsForList();
listOperations.leftPushAll(key, "111", "222", "333");
List range = listOperations.range(key, 0, listOperations.size(key));
return JSON.toJSONString(range);
}
@RequestMapping("/opsForSet")
public String opsForSet(String key) {
SetOperations setOperations = redisTemplate.opsForSet();
setOperations.add(key, "aaa");
setOperations.add(key, "bbb");
Set members = setOperations.members(key);
return JSON.toJSONString(members);
}
@RequestMapping("/opsForZSet")
public String opsForZSet(String key) {
ZSetOperations zSetOperations = redisTemplate.opsForZSet();
zSetOperations.add(key, "aaa", 1);
zSetOperations.add(key, "bbb", 2);
zSetOperations.add(key, "ccc", 3);
Set range = zSetOperations.range(key, 0, zSetOperations.size(key));
return JSON.toJSONString(range);
}
3.4 BoundXxxOperations 方式
@RequestMapping("/boundValueOps")
public String boundValueOps(String key, String value) {
BoundValueOperations boundValueOps = redisTemplate.boundValueOps(key);
boundValueOps.set(value);
Object obj = boundValueOps.get();
return obj.toString();
}
@RequestMapping("/boundHashOps")
public String boundHashOps(String key, String hashKey, String value) {
BoundHashOperations boundHashOperations = redisTemplate.boundHashOps(key);
boundHashOperations.put(hashKey, value);
boundHashOperations.put(hashKey, value + "1");
Set keys = boundHashOperations.keys();
return JSON.toJSONString(keys);
}
@RequestMapping("/boundListOps")
public String boundListOps(String key) {
BoundListOperations boundListOperations = redisTemplate.boundListOps(key);
boundListOperations.leftPush("111");
boundListOperations.leftPush("222");
boundListOperations.rightPush("333");
boundListOperations.rightPush("444");
List range = boundListOperations.range(0, boundListOperations.size());
Object index = boundListOperations.index(1);
Object obj = boundListOperations.leftPop();
return JSON.toJSONString(range);
}
@RequestMapping("/boundSetOps")
public String boundSetOps(String key) {
BoundSetOperations boundSetOperations = redisTemplate.boundSetOps(key);
boundSetOperations.add("111");
boundSetOperations.add("222");
boundSetOperations.add("333");
Set members = boundSetOperations.members();
return JSON.toJSONString(members);
}
@RequestMapping("/boundZSetOps")
public String boundZSetOps(String key) {
BoundZSetOperations boundZSetOperations = redisTemplate.boundZSetOps(key);
boundZSetOperations.add("111", 1);
boundZSetOperations.add("222", 2);
boundZSetOperations.add("333", 3);
Set range = boundZSetOperations.range(0, boundZSetOperations.size());
return JSON.toJSONString(range);
}
【信息由网络或者个人提供,如有涉及版权请联系COOY资源网邮箱处理】
© 版权声明
部分内容为互联网分享,若有侵权请联系站长删除。
THE END
暂无评论内容