1. 通用工具
入口 http://localhost:8080/tool
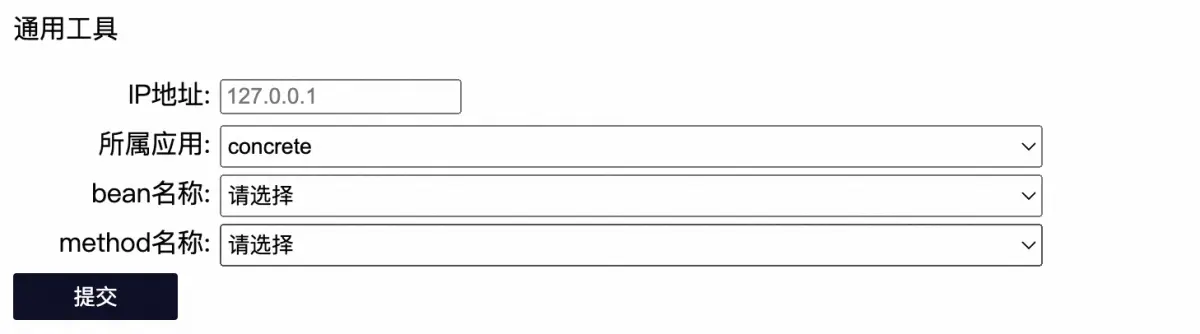
执行结果:
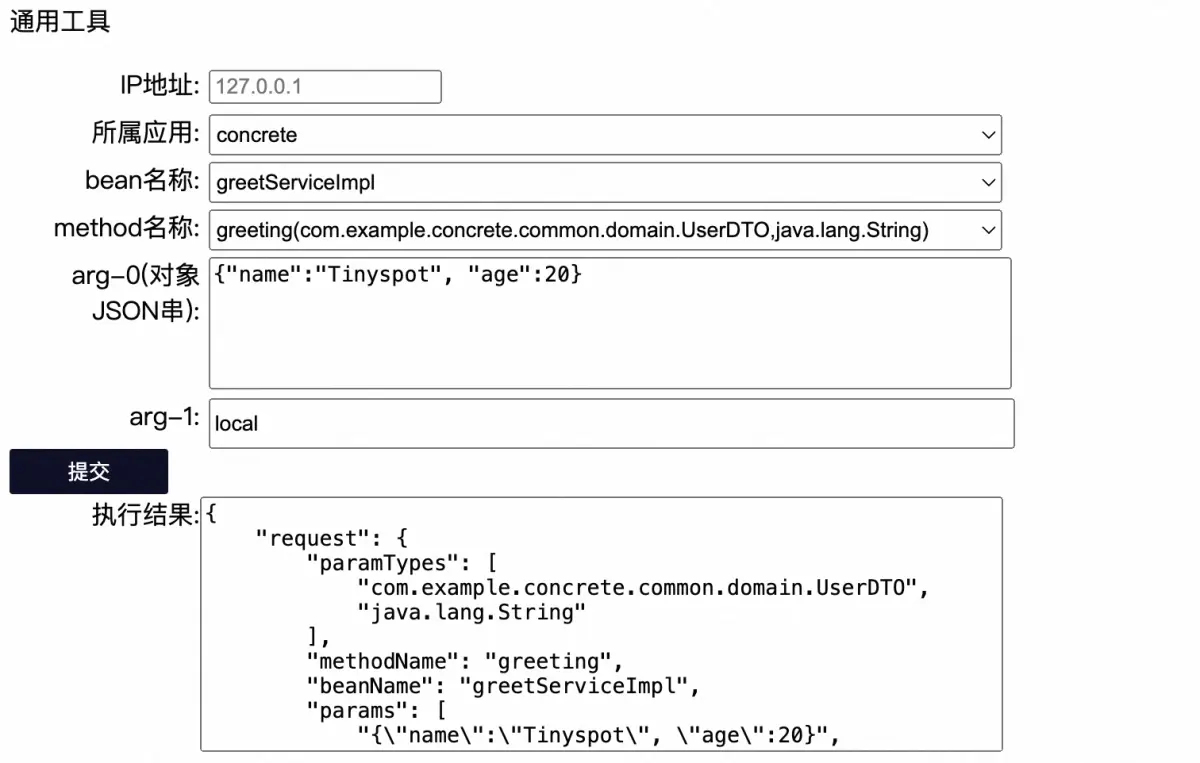
2. 获取所有Bean
@Controller
@RequestMapping("tool")
public class BeanTools {
@GetMapping(value = {"", "/common"})
public ModelAndView common() {
ModelAndView modelAndView = new ModelAndView();
modelAndView.addObject("beanNames", getBeanNames());
modelAndView.setViewName("invoke.html");
return modelAndView;
}
private List getBeanNames() {
List beanNames = SpringBeanUtils.getBeans();
List beanObjs = new ArrayList();
beanNames.forEach(str -> {
JSONObject obj = new JSONObject();
obj.put("text", str);
obj.put("value", str);
beanObjs.add(obj);
});
return beanObjs;
}
}
@Component
public class SpringBeanUtils implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
SpringBeanUtils.applicationContext = applicationContext;
}
public static ApplicationContext getApplicationContext() {
return applicationContext;
}
public static List getBeans() {
String[] beanDefinitionNames = getApplicationContext().getBeanDefinitionNames();
List beanNames = Arrays.stream(beanDefinitionNames)
.filter(str -> !str.contains("."))
.collect(Collectors.toList());
beanNames.sort(String::compareTo);
return beanNames;
}
}
3. 获取 Bean 的方法
@RestController
@RequestMapping("/ajax")
public class BeanAjax {
@GetMapping("/getMethods")
public List- getMethods(String beanName) {
return getBeanMethods(beanName);
}
@PostMapping("/beanMethods")
public List
- beanMethods(AjaxPojo ajaxPojo) {
return getBeanMethods(ajaxPojo.getBeanName());
}
private List
- getBeanMethods(String beanName) {
List
- list = new ArrayList();
Object bean = SpringBeanUtils.getBean(beanName);
Method[] methods = bean.getClass().getMethods();
for (Method method : methods) {
String methodSign = method.toString();
String paramSign = methodSign.substring(methodSign.indexOf("("), methodSign.indexOf(")") + 1);
list.add(new Item(method.getName() + paramSign, method.getName() + paramSign));
}
return list;
}
@Data
public class Item implements Serializable {
private static final long serialVersionUID = -6504357622310055548L;
private String text;
private String value;
public Item(String text, String value) {
this.text = text;
this.value = value;
}
}
@Data
public class AjaxPojo implements Serializable {
private String appName;
private String beanName;
private String methodName;
private List
args = new ArrayList();
}
}
4. 执行方法
@RestController
@RequestMapping("/ajax")
public class BeanAjax {
@PostMapping("/invoke")
public JSONObject invoke(AjaxPojo ajaxPojo) throws InvocationTargetException, IllegalAccessException {
String beanName = ajaxPojo.getBeanName();
String methodName = ajaxPojo.getMethodName();
List args = ajaxPojo.getArgs();
Object bean = SpringBeanUtils.getBean(beanName);
Method method = findMethod(bean, methodName);
Object invoke = method.invoke(bean, ReflectDemo.assemblyArgs(args, method.getParameterTypes()));
JSONObject result = new JSONObject();
JSONObject request = new JSONObject();
request.put("beanName", beanName);
request.put("methodName", method.getName());
request.put("params", ajaxPojo.getArgs());
request.put("paramTypes", method.getParameterTypes());
JSONObject response = new JSONObject();
response.put("data", invoke);
response.put("success", "true");
result.put("request", request);
result.put("response", response);
return result;
}
private Method findMethod(Object bean, String methodName) {
Method[] methods = bean.getClass().getMethods();
for (Method method : methods) {
String methodSign = method.toString();
if (methodSign.contains(methodName)) {
return method;
}
}
return null;
}
@Data
public class AjaxPojo implements Serializable {
private String appName;
private String beanName;
private String methodName;
private List args = new ArrayList();
}
}
public class ReflectDemo {
/**
* 参数组装
*
* @param arguments 参数值
* @param parameterTypes 参数类型
* @return
*/
public static Object[] assemblyArgs(List arguments, Class>[] parameterTypes) {
List
5. 页面 invoke.html
Invoke
通用工具
【信息由网络或者个人提供,如有涉及版权请联系COOY资源网邮箱处理】
© 版权声明
部分内容为互联网分享,若有侵权请联系站长删除。
THE END
暂无评论内容